BoxPay Android SDK enables swift creation of a payment process within your Android application. We offer robust and adaptable UI components ready for immediate use, allowing you to effortlessly accept payments from your shoppers.
To begin integrating with the BoxPay Checkout, the initial step is to register and setup your BoxPay account.
This outlines the typical workflow for integrating a BoxPay Android checkout SDK –
- The shopper taps on the Buy/Pay button on the Merchant App.
- The Merchant Server processes the request and initiates a checkout session through the Create Checkout Session API.
- The Merchant Server receives the BoxPay Checkout token in the response and returns it back to Merchant App.
- Merchant App initiates the BoxPay payment flow by invoking Checkout SDK. Please refer to the Android Checkout SDK Integration Steps section for more details around it.
- The shopper completes the payment on the BoxPay Payment Sheet.
- After payment, BoxPay calls the result callback method with the payment status.
- Depending on the payment status received, the Merchant App redirects the customer to either the Order Confirmation page, or to the payment failed/canceled page.
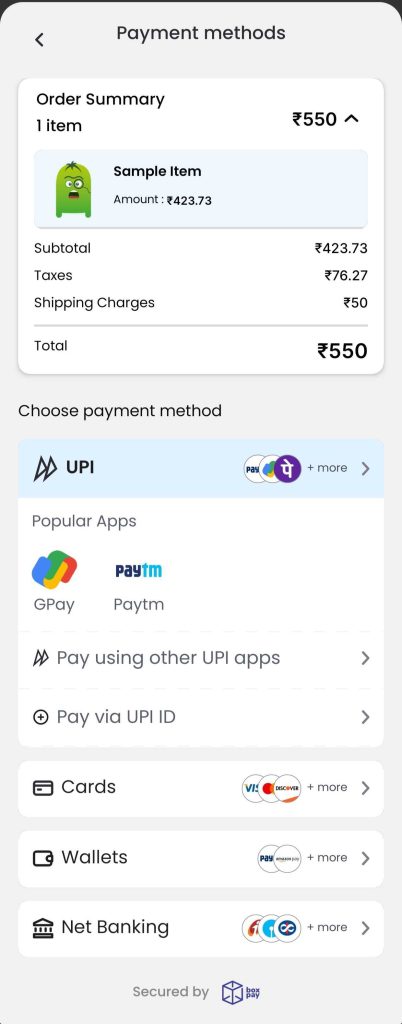
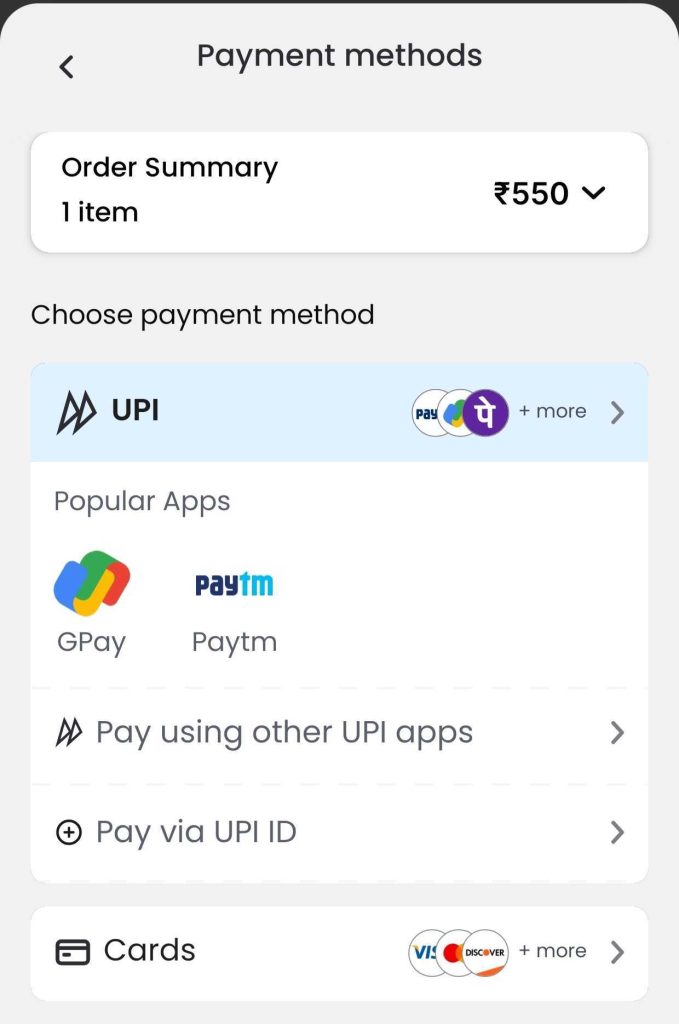
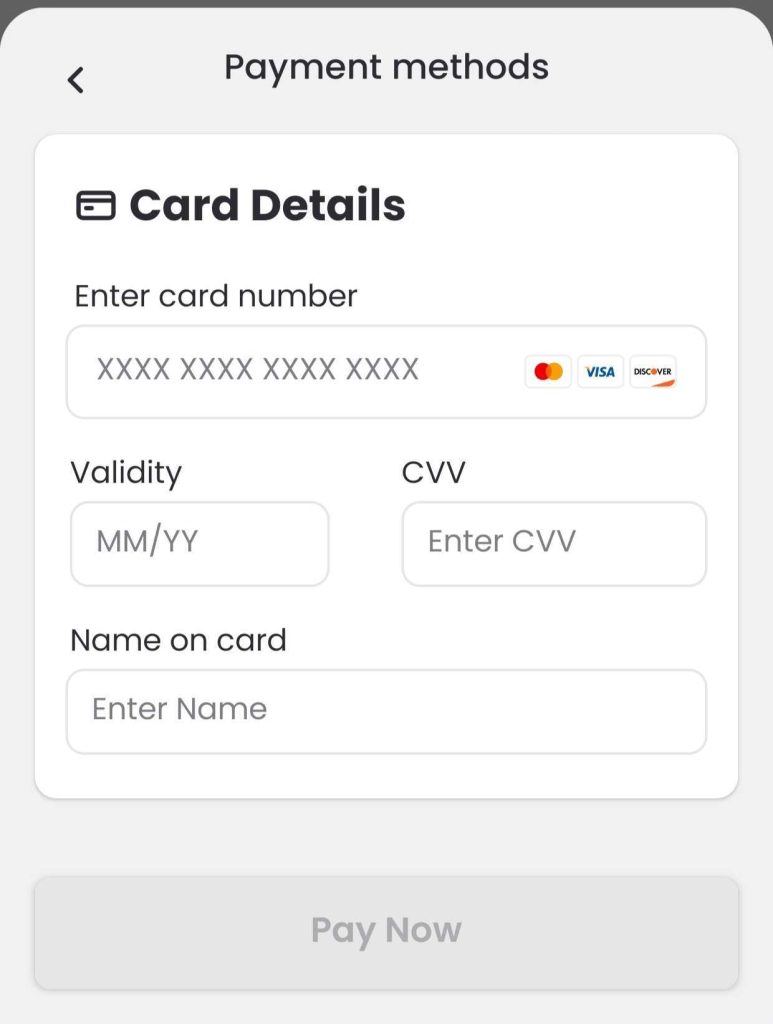
Android Checkout SDK Integration Steps
Add Maven repository
To start with, you need to add following Jitpack Maven repository to repositories block in your build.gradle/settings.gradle file –
repositories {
// …
maven { url = uri("https://www.jitpack.io") }
}
Add SDK dependency
Next step is to add the SDK dependency in your build.gradle (module: app) to access the SDK classes in your App –
plugins {
id("com.android.application")
}
android { ... }
dependencies {
// ...
// BoxPay Android SDK
implementation("com.github.BoxPay-SDKs:checkout-android-sdk:1.2.0")
}
Initiate the SDK payment flow
In order to initiate the payment flow, you need to create a checkout session from your server endpoint. Additionally, you need to pass the payment result callback function to get notified when the payment is either successful or cancelled by the user. In case of failures, we prompt user to retry in the BoxPay payment sheet itself.
Finally, you can initiate the payments flow using the SDK classes as shown below –
Kotlin Sample Code –
fun initiatePayment() {
val checkoutToken =
""// Call your server API that will in-turn call the BoxPay create checkout session api to get the checkout token
val shopperToken = ""//From the same api call in payload response shopper_token will be received
val boxPayCheckout = BoxPayCheckout(
context = this,
token = checkoutToken,
onPaymentResult = ::onPaymentResultCallback,
customerShopperToken = shopperToken,
configurationOptions = mapOf(
ConfigurationOptions.SHOW_UPI_QR_ON_LOAD to true, // this is for QR related changes, to show QR as the checkout loads send its value as true as shown -
ConfigurationOptions.ENABLE_SANDBOX_ENV to true, // for sandbox env, pass its value as true as shown -
ConfigurationOptions.SHOW_BOXPAY_SUCCESS_SCREEN to true // to show Boxpay success screen pass its value as true as shown -
)
)
// if you don't want to pass anything then simply pass as null and at that time it will be prod env by default as shown below -
// val boxPayCheckout = BoxPayCheckout(
// context = this,
// token = checkoutToken,
// onPaymentResult = ::onPaymentResultCallback,
// customerShopperToken = shopperToken,
// configurationOptions = null
// )
boxPayCheckout.display()
}
fun onPaymentResultCallback(result : PaymentResultObject) {
if (result.status == "Success") {
// redirect to success screen
} else {
// Handle other payment statuses
}
}
//here the PaymentResultObject is:
class PaymentResultObject(private val resultFetched : String,private val transactionIdFetched : String) {
var status : String ?= null
var transactionId : String ?= null
init {
this.status = resultFetched
this.transactionId = transactionIdFetched
}
}
Java Sample Code –
public void initiatePayment() {
String checkoutToken = "";
// Call your server API that will in-turn call the BoxPay create checkout session API to get the checkout token
String shopperToken = "";
// From the same API call in payload response shopper_token will be received
BoxPayCheckout boxPayCheckout = new BoxPayCheckout(
this,
checkoutToken,
this::onPaymentResultCallback, // Assuming you have a method named onPaymentResultCallback
shopperToken,
new HashMap<String, Object>() {{
put(ConfigurationOptions.SHOW_UPI_QR_ON_LOAD, true); // This is for QR-related changes, to show QR as the checkout loads
put(ConfigurationOptions.ENABLE_SANDBOX_ENV, true); // for sandbox env, pass its value as true as shown -
put(ConfigurationOptions.SHOW_BOXPAY_SUCCESS_SCREEN, true); // to show Boxpay success screen pass its value as true as shown -
}}
);
// If you don't want to pass anything, then simply pass as null, and it will default to the production environment:
/*
BoxPayCheckout boxPayCheckout = new BoxPayCheckout(
this,
checkoutToken,
this::onPaymentResultCallback,
shopperToken,
null
);
*/
boxPayCheckout.display();
}
public Unit onPaymentResultCallback(PaymentResultObject result){
if (result.status == "Success") {
// redirect to success screen
} else {
// Handle other payment statuses
}
return Unit.INSTANCE;
}
//here the PaymentResultObject is:
class PaymentResultObject(private val resultFetched : String,private val transactionIdFetched : String) {
var status : String ?= null
var transactionId : String ?= null
init {
this.status = resultFetched
this.transactionId = transactionIdFetched
}
}
Verifying the payment status
Once you get the PaymentResultObject in your callback function, you can verify/handle the status as below:
Status | Description |
---|---|
Success | Payment was received successfully. You can proceed to order fulfilment. It is equivalent to Approved status received in Operation Inquiry API response. |
NoAction | Shopper didn’t attempt the payment at all. No transactionId or operationId will be received in this case. |
RequiresAction | Shopper initiated the payment but didn’t complete the payment by entering the OTP, scanning QR code, approving collecting request etc. based on the chosen payment method. |
Processing | Shopper completed the payment but PSP didn’t provide the approval confirmation. It may be due to additional risk checks or merchant account issues. You should not proceed to order fulfilment and rather wait for the terminal status(Approved or Failed/Rejected) via webhooks. It is equivalent to Posted status received in Operation Inquiry API response. |
Failed | Shopper attempted the payment but it was failed. Shopper may have made multiple attempts as we prompt used for retries. You will be provided with latest operationId along with transactionId. It is equivalent to Failed and Rejected status received in Operation Inquiry API response. |
Getting the payment details using Operation Inquiry endpoint
If you need to get additional details, you can invoke BoxPay Operation Inquiry API from your server side component using the transactionId and operationId received in PaymentResultObject as you must NOT store the BoxPay API key in the Android App.